Android Native SDK
Comprehensive reference of iZooto's SDK methods for Android apps built with the Native framework.
Just starting with Android?
Check out our Android Native SDK Integration guide.
Parameter | Data Type | Description |
---|---|---|
Initialisation | ||
init | Builder Method | Initialises iZooto to register the device for push notifications. Should be called in the onCreate method of your Application class. See Native SDK Setup for details and code examples. |
setTokenReceivedListener | Builder Method | Includes the onTokenReceived method to get the subscription token for the current device. |
setNotificationReceiveListener | Builder Method | Includes the onNotificationReceived and onNotificationOpened methods to send and receive data when notification is received or clicked. |
setLandingURLListener | Builder Method | Includes the onWebView method to open Landing URL inside your app's activity. |
unsubscribeWhenNotificationsAreDisabled | Builder Method | If notifications are disabled for your app, unsubscribe them from iZooto. |
Notification Channel | ||
setNotificationChannelName | Method | Can be used to set a custom channel name for the notifications. |
User Permission | ||
setSubscription | Method | Disables iZooto from sending notifications to the current device. |
navigateToSettings | Method | Can be used to re-direct the users to the App Settings page to check the notification permissions, etc. |
Sound | ||
setNotificationSound | Method | Customize the sound that gets played whenever a notification is received on the device. |
Analytics | ||
setFirebaseAnalytics | Method | Send events to Google Analytics to track your campaign performance. |
Android Tags | ||
addTag | Method | Use this method to tag a user to a specific value. |
removeTag | Method | Use this method to remove already tagged users from a value. |
Pulse | ||
enablePulse | Method | Use this method to show a personalised news feed to your app users. |
Google One Tap | ||
requestOneTap | Method | Use this method to show the Google One Tap prompt to your app users. |
Get Tags List REST: GET API | API | Get a list of all the tags that you have created for your app along with the number of users inside that tag. |
Send Campaigns to Tagged Users REST: POST API | API | Use this to send out notifications to only your tagged users. |
Initialization
init
init
Initialises iZooto to register the device for push notifications. Should be called in the onCreate
method of your Application class. See Native SDK Setup for details and code examples.
iZooto.initialize(this)
.build();
Overloaded function accepts previously generated token by FCM, Xiaomi or Huawei. When the correct JSON is provided, the SDK doesn't request cloud messaging service for the token. If the JSON is invalid or the token is of incorrect length, the SDK will generate a new token.
Note: Developers need to make sure that the token passed is valid and active, or the device will be unable to receive notifications.
iZooto.initialize(this, String tokenJson)
.build();
The parameter tokenJson
is the JSON that contains tokens by the respective cloud messaging service. The JSON may contain both FCM and Xiaomi tokens for the same device.
{
"fcmToken":"<FCM TOKEN>",
"xiaomiToken":"<XIAOMI TOKEN>",
"huaweiToken":"<HUAWEI TOKEN>"
}
setTokenReceivedListener
setTokenReceivedListener
This method will return the subscription token of the current device. The token would only be visible when the app has been launched after installation.
Sample code:
iZooto.initialize(this)
.setTokenReceivedListener(this)
.build();
@Override
public void onTokenReceived (String token) {
Log.i("Device Token: ", token + "");
}
setNotificationReceiveListener
setNotificationReceiveListener
The instance contains two methods that will be called either when a notification is received or when a notification is tapped on from the notification shade.
Sets notification opened and received handler.
Parameter | Type | Description |
---|---|---|
Method | onNotificationReceived | Called when a notification is received by your Android device. |
Method | onNotificationOpened | Called when a notification is tapped on. Used to open any specific activity on notification click. |
Sample code:
// INITIALIZATION
iZooto.initialize(this)
.setNotificationReceiveListener(new ExampleNotificationHandler())
.build();
// METHOD CALL
class ExampleNotificationHandler implements NotificationHelperListener {
@Override
// FIRES WHEN A NOTIFICATION IS RECEIVED
public void onNotificationReceived (Payload payload) {
Log.i("iZootoExample", "payload = " + payload.getTitle());
}
/*
1. If we clicked notification, then it will show actionType 0.
2. If we clicked button 1, then it will show actionType 1.
3. If we clicked button 2, then it will show actionType 2. */
// FIRES WHEN A NOTIFICATION IS CLICKED
public void onNotificationOpened (String data) {
String actionType;
try {
JSONObject jsonObj = new JSONObject(data);
actionType = jsonObj.String("actionType");
if (actionType.equalsIgnoreCase("0")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
} else if (actionType.equalsIgnoreCase("1")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
} else if (actionType.equalsIgnoreCase("2")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
// INITIALIZATION
iZooto.initialize(this)
.setNotificationReceiveListener(ExampleNotificationHandler())
.build()
companion object {
lateinit var instance: MyApplication
private set
}
// METHOD CALL
internal class ExampleNotificationHandler : NotificationHelperListener {
// FIRES WHEN A NOTIFICATION IS RECEIVED
override fun onNotificationReceived(payload: Payload) {
Log.i("iZootoExample", "payload = " + payload.title)
}
/*
1. If we clicked on notification then, it will show actionType 0.
2. If we clicked on button 1 then, it will show action type 1.
3. If we clicked on button 2 then, it will show action type 2.*/
// FIRES WHEN NOTIFICATION IS CLICKED
override fun onNotificationOpened(data: String) {
var actionType: String? = null
try {
val jsonObj = JSONObject(data)
actionType = jsonObj.getString("actionType")
if (actionType.equals("0")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
} else if (actionType.equals("1")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
} else if (actionType.equals("2")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
}
} catch (e: JSONException) {
Log.e("TAG", "notificationOpened: " + e);
e.printStackTrace();
}
}
}
setLandingURLListener
setLandingURLListener
This method can be used to override iZooto's Landing URL and directly open them inside your app's WebView. No explicit notification handler code is required and you would not have to define additional key: value
pairs to do so.
Using this will significantly reduce the campaign creation time.
Sample code:
// INITIALIZATION
iZooto.initialize(this)
.setLandingURLListener (new ExampleNotificationWebViewHandler())
.build();
// METHOD CALL
class ExampleNotificationWebViewHandler implements NotificationWebViewListener {
// TO OPEN LANDING URL INSIDE YOUR ACTIVITY
@Override
public void onWebView (String landingUrl) {
if (landingUrl!=null) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.putExtra("openURL", landingUrl);
startActivity(intent);
}
}
}
// INITIALIZATION
iZooto.initialize(this)
.setLandingURLListener (new ExampleNotificationWebViewHandler())
.build()
//METHOD CALL
internal class ExampleNotificationWebViewHandler : NotificationWebViewListener {
/*To open landing url inside your activity*/
override fun onWebView(landingUrl: String) {
if (landingUrl != null) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
intent.putExtra("landingUrl", landingUrl)
instance.startActivity(intent)
}
}
}
unsubscribeWhenNotificationsAreDisabled
unsubscribeWhenNotificationsAreDisabled
Android Devices can still get Data/Silent Notifications even if the user unsubscribes from the App Settings. This will happen when your users go to Settings > Apps and turn off notifications or if they long-press one of your notifications and select "block notifications".
If notifications are disabled for your app, setting this method to true will unsubscribe the user from iZooto and re-subscribe them if they turn notifications back on.
This is false by default, so Android users will be marked as subscribed in this case and can receive data/silent notifications. They will not see any data or get alerted if they unsubscribe from the App Settings.
Parameter | Type | Description |
---|---|---|
prompt | Boolean | false (Default) - don't unsubscribe userstrue - unsubscribe users when notifications are disabled |
iZooto.initialize(this)
.unsubscribeWhenNotificationsAreDisabled(true)
.build();
iZooto.initialize(this)
.unsubscribeWhenNotificationsAreDisabled(true)
.build()
Notification Channel
setNotificationChannelName
setNotificationChannelName
This method can be used to define a custom Notification Channel name for the push notifications.
setNotificationChannelName(String channelName);
Points to Note
- The Notification Channel name cannot be more than 30 characters and the name cannot contain special characters.
- If no channel name is provided or if the character length is more than 30 characters, then the channel name will be
AppName Notifications
.- If the App Name is also not available, then the channel name will be
Push Notifications
.
User Permission
setSubscription
setSubscription
Can be used to not deliver notifications to a specific device. This method does not officially subscribe or unsubscribe users from the app settings, it unsubscribes them from receiving a push from iZooto.
You can call this method with false
to opt-out users from receiving notifications through iZooto. You can pass true
later to opt users back into notifications.
iZooto.setSubscription(true);
iZooto.setSubscription(true)
Please make sure that the application class is defined in your AndroidManifest.XML file.
navigateToSettings
navigateToSettings
This method can be used to take the users to the App Settings page to check the notification permissions and/or other app settings. This method becomes useful if the user has disabled notifications and you would like to have an option inside the app itself to re-direct the user to the App Settings page to allow the user to re-enable the notification permissions. The method can be called at the click of a button or any other event as per requirement.
navigateToSettings(Activity activity)
Sound
setNotificationSound
setNotificationSound
Used to set a custom sound to the notifications when delivered on the device. The device's usual notification sound is played by default, which can be overridden to use a custom sound for your app.
Inside Application Class:
iZooto.setNotificationSound("Sound_File_Name");
Points to Note
- The notifications sound file should already be present in the
res/raw
folder of the project.- Supported file types are .mp3 and .wav.
- The file name needs to be passed without the file extension.
Analytics
setFirebaseAnalytics
setFirebaseAnalytics
iZooto will automatically send notification events to your analytics dashboard if Google Analytics for Firebase is correctly implemented.
Events
The iZooto SDK tracks events that pertain to notification open & receive events. The following events are sent:
Event Name | Purpose |
---|---|
push_notification_opened | An iZooto notification was opened |
push_notification_received | An iZooto notification was received. |
push_notification_influence_open | An application was opened within 2 minutes of an iZooto notification being received. |
The iZooto SDK also sends parameters that contain more info about the particular notification the event is attributed to. These can be directly defined when you create a campaign.
Parameter Name | Purpose |
---|---|
source | To attribute this event's source to the iZooto SDK |
medium | A formal indication that the medium for the event is a notification. |
campaign | By default, it would be your campaign name. |
term | Can be used to track keywords |
content | Can be used to differentiate between links that point to the same URL. |
Follow the Firebase integration documentation and verify that Firebase is correctly functioning inside your application.
Once everything is set up, use the following method to enable passing events to Firebase.
iZooto.setFirebaseAnalytics(true);
iZooto.setFirebaseAnalytics(true)
You're Done!
Google Analytics for Firebase is now set up to receive iZooto events.
Pulse
enablePulse
enablePulse
Pulse is a personalized news feed that can be embedded within the app.
Below is the list of required parameters.
Parameter Name | Purpose |
---|---|
activityObject | Pass the current activity object. |
coordinatelayoutId , linearlayoutId | Pass the coordinate or the linear layout ID from the layout. |
scrollViewId | Pass the scroll view ID (only needed if you are using linear layout) |
isProgressBar | true /false - true enables the Progress Bar, false disables it. |
Syntax for Coordinate Layout
iZooto.enablePulse(<activityObject>, <coordinatelayoutId>, <isProgressBar>);
Example
iZooto.enablePulse(this, mainLayout, true)
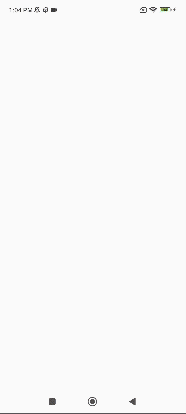
Syntax for Linear Layout
iZooto.enablePulse(<currentObject>, <scrollViewId>, <linearLayoutId>, <isProgressBarEnable>)
Example
iZooto.enablePulse(this, customScroll, customLayout, true)
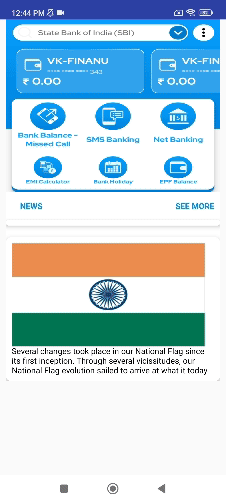
Google One Tap
requestOneTap
requestOneTap
If you do not have Google One Tap enabled in your app, use the below method in your main Activity class:
iZooto.requestOneTapActivity(<ActivityContext>, new OneTapCallback() {
@Override
public void syncOneTapResponse(String email, String fName, String lName) {
Log.e("Email >> ",email);
Log.e("First_Name >> ",fName);
Log.e("Last_Name >> ",lName);
}
});
If you already have Google One Tap enabled in your app, use the below method to pass the information to iZooto:
iZooto.syncUserDetailsEmail(<context>,"your_email", "your_firstName","your_lastName");
Updated 10 months ago
See other integrations you can set up with iZooto