Native Android Troubleshooting
Troubleshooting common issues with our Native Android SDK.
Troubleshooting Steps
Check the below first for common issues with Native Android Setup.
Try our example project on GitHub.
If stuck, contact support directly by sending an email to [email protected].
For faster assistance, please provide the following:
- Your iZooto App ID (available under Settings > General > Account ID for the Android property on the panel)
- Details, logs, and screenshots of the issue
- Steps to reproduce
Ensure you follow all the steps mentioned below. If you are still facing issues, please reach out to Support with an error log generated using the steps given below.
Step 1: Double Check Setup Guide
Return to the setup guide you followed in Android Native SDK Setup to make sure you followed all steps while adding the iZooto SDK to your app.
Step 2: Initialise within the Application Class
Make sure you added the iZooto init
within the onCreate
method in your Application
class.
Step 3: Check below for common issues, or send us a log if still not sure
After running through the rest of this Troubleshooting doc, share the crash logs with our support team for us to understand and assist you better.
ANR Troubleshooting
ANR errors occur when the main thread of your app is being blocked for over 5 seconds. You may see these occur along with:
Broadcast of Intent { act=com.google.android.c2dm.intent.RECEIVE flg=0x11000010 pkg=YOUR.PACKAGE.NAME cmp=your.package.name/com.iZooto.FCMBroadcastReceiver (has extras) }
This just shows how the app process started. This may appear to occur more often after adding iZooto due to push notification received events starting your app process.
The best way to dig into these ANR issues is to check the full stacktrace of all threads.
Starting at the top of each stacktrace and working down, it will help guide you on which event occurred last to trigger the ANR.
If you see iZooto in the actual stacktrace, please make sure you are testing on the latest version of our SDK. Once you verify that the issue still persists, send the full stacktrace of all threads with the ANR to [email protected] and we will help investigate.
The full log would most likely be in the low 1,000s of lines long.
Why are notifications disappearing without clicking them?
Check if you are using the Native Android method NotificationManagerCompat.from(context).cancelAll()
.
Failed to resolve: com.izooto:android-sdk:[1.0.0,2.1.9]
This means that Android Studio or Gradle could not download our plugin. Please check the following:
1. Open your browser to https://search.maven.org to make sure it loads on your system.
2. Make sure you are using Android Studio version 1.4.0 or newer.
3. Go to File > Settings
.
4. Search for Offline work and uncheck this option.
5. Add the following to your .gradle
file.
repositories {
mavenCentral()
}
6. Try restarting Android Studio and then going to Tools > Android > Sync Project with Gradle files
.
Error: Execution failed for task ':app:processDebugGoogleServices'
If you are receiving the following Android Studio error when building your project
Error:Execution failed for task ':app:processDebugGoogleServices'.
> Please fix the version conflict either by updating the version of the google-services plugin (information about the latest version is available at https://bintray.com/android/android-tools/com.google.gms.google-services/) or updating the version of com.google.android.gms to 9.0.0.
Remove the following line from your .gradle
file.
apply plugin: 'com.google.gms.google-services'
E/dalvikm: Could not find class
Could not find class errors are expected in the logcat for Android devices before 5.0. It is just letting you know it could not find these classes as your app loads into memory. It does not cause any issues in your app as the calls are guarded with runtime checks so they won't create any issues.
Error: java.lang.NoSuchMethodError: com.google.android.gms.common.internal.zzaa.zzb
If you see that some obfuscated Firebase or Google GMS methods are missing, it is most probably a dependency versioning conflict.
You can use the gradle dependencies
and gradle dependencyInsight
directives to troubleshoot which libraries are causing classes/methods to go missing. Refer to the official Gradle documentation for more information:
https://docs.gradle.org/current/userguide/tutorial_gradle_command_line.html#sec:dependency_insight
For example:
./gradlew app:dependencyInsight --configuration compile
Error:Execution failed for task ':app:processDebugManifest'
If you see the following error make sure you have completed step 2 of the Android Native Setup correctly.
Execution failed for task ':app:processDebugManifest'
Manifest merger failed with multiple errors, see logs
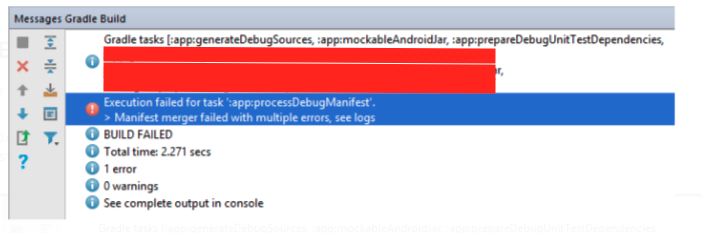
Error:(3,0) startup failed: only buildscript {} and other plugins {} script blocks are allowed before plugins {} blocks, no other statements are allowed
Make sure you added the code from step 2 to the very top of the build.gradle
file. This should be the first line of code in the file.
ERROR: AppId format is invalid
1. Make sure you have izooto_app_id
in your build.gradle
file and the id is correct.
android {
defaultConfig{
manifestPlaceholders = [
izooto_app_id : 'YOUR_iZOOTO_ACCOUNT_ID_HERE'
]
}
}
The
izooto_app_id
is available under Settings > General.
2. Make sure you are not replacing the application
tag in your AndroidManifest.xml
file with tools:node="replace"
<application
android:icon="@mipmap/ic_launcher"
tools:node="replace" <!-- Remove this line!!! -->
android:name=".ApplicationClass">
If you must replace some attributes, please use tools:replace
instead of tools:node
.
Example: tools:replace="icon, label"
Error: Missing Google Play Services Library
In Android Studio, open build.gradle (Module: app)
and make sure you are using the latest iZooto SDK under dependencies.
dependencies {
implementation 'com.izooto:android-sdk:[1.0.0,2.1.9]'
}
How to get a crash or error log from an Android device
With Android Studio
1. Select Android Monitor
from the bottom of the window.
------If you don't see this option, select it from View > Tool Windows > Android Monitor
2. Select your device from the drop down.
3. Ensure no filters are set and the type is set to Verbose.
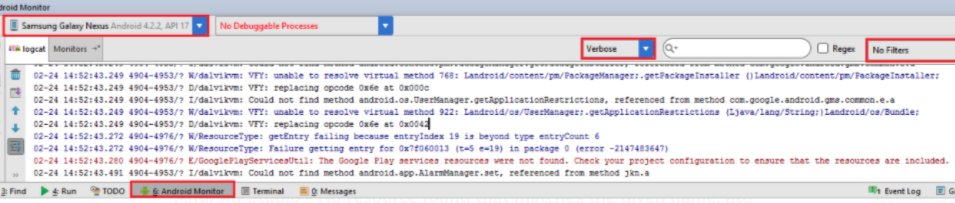
4. Select all lines in the log by pressing Control + A and then copy them.
5. Paste them into a .txt
file and send this to our support team. Include steps to reproduce the problem as well.
Android Studio - No resource found that matches the given name: attr 'android:keyboardNavigationCluster'
Make sure you have compileSdkVersion
to 26
in the app/build.gradle
. This is required when you update to 26 of the Android Support Library.
Eclipse - ERROR - "conversion to dalvik format failed with error 1"
If you are getting a conversion to dalvik format failed with error 1
error with Dx bad class file magic (cafebabe) or version (0033.0000)
messages before this, then you may have the wrong Java version set on your system. See the below post to fix this as well as the other answers.
http://stackoverflow.com/a/9041471/1244574
Updated almost 3 years ago