Call to Action Buttons
Additional configurable actions that users may take on notifications.
Typically when a notification is received, there is only a single action available - tapping on the notification. However, the Call to Action buttons allow more than one action to be taken on a notification, allowing for greater user interaction within the notifications.
How to Add Call to Action Buttons from the Panel
While Creating a Campaign for app push, under the Message field, you can enable the CTA's toggle to add Call to Action buttons for your notifications.
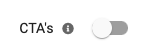
Once enabled, you will see the option to input details for your call to action buttons.
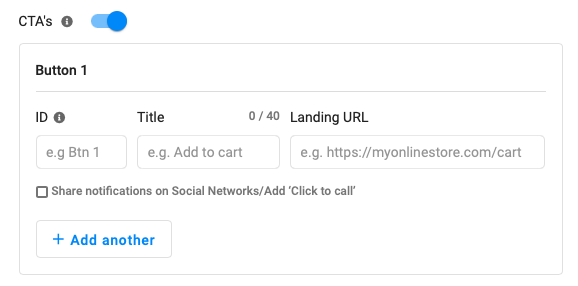
You can add another call to action button by clicking on + Add Another.
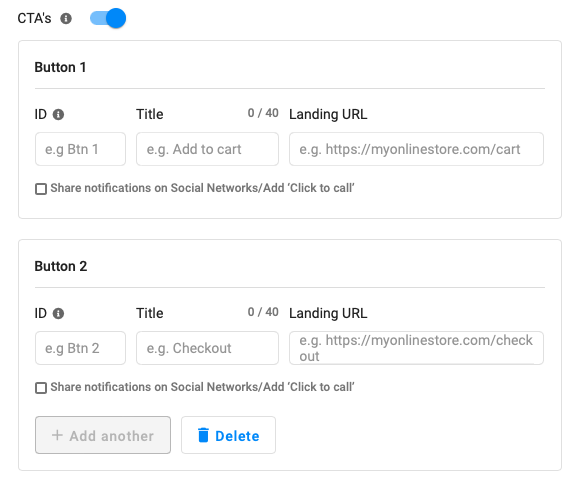
You can add a maximum of 2 buttons. This is to ensure that the notification style is kept intact and is visible on all devices properly.
You can also add call to action buttons to share the notification on social media channels. Currently, we provide support for Facebook, Twitter, and LinkedIn.
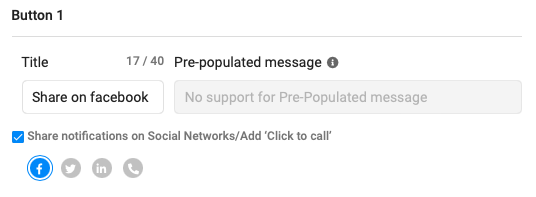
Fields to be Added when Enabling Call to Action Buttons
The below fields need to defined for every call to action button. This can be done from the panel as well as using our Rest APIs.
Dashboard | API Parameter | Notes |
---|---|---|
ID | id | A unique identifier for your call to action button. The ID of the clicked button is passed to you so that you can identify which button was clicked. |
Title | text | The text visible on the CTA. Passed to your app so you can identify which button was clicked. |
Landing URL | url | The URL to open when the CTA is clicked. This can be configured to open any activity inside the app as well. |
How to Add Action Buttons using our Rest API and SDK
1. Rest API
Please refer to our Push APIs guide for help on how to define call to action buttons using our Rest APIs for both Android and iOS. The button ID is passed with the deep links data for configuration at your end.
2. SDK
Please see our SDK References for App Push document for whichever SDK you are using to build your app. The button ID is passed with the deep links data for configuration at your end.
How to Handle the Call to Action Button Click Event
When a call to action button is clicked, the iZooto SDK fires a notification click event also known as the Notification Opened Handler.
Inside these click events, you can check for the ActionType id
which you set when creating the call to action buttons. If the id
is available, you can then perform some additional action within this event handler like deep links or another custom process.
// INITIALIZATION
iZooto.initialize(this)
.setNotificationReceiveListener(new ExampleNotificationHandler())
.build();
// METHOD CALL
class ExampleNotificationHandler implements NotificationHelperListener {
@Override
// FIRES WHEN A NOTIFICATION IS RECEIVED
public void onNotificationReceived (Payload payload) {
Log.i("iZootoExample", "payload = " + payload.getTitle());
}
/*
1. If we clicked notification, then it will show actionType 0.
2. If we clicked button 1, then it will show actionType 1.
3. If we clicked button 2, then it will show actionType 2. */
// FIRES WHEN A NOTIFICATION IS CLICKED
public void onNotificationOpened (String data) {
String actionType;
try {
JSONObject jsonObj = new JSONObject(data);
actionType = jsonObj.String("actionType");
if (actionType.equalsIgnoreCase("0")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
} else if (actionType.equalsIgnoreCase("1")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
} else if (actionType.equalsIgnoreCase("2")) {
Intent intent =new Intent(getApplicationContext(), YOURACTIVITY.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
// INITIALIZATION
iZooto.initialize(this)
.setNotificationReceiveListener(ExampleNotificationHandler())
.build()
companion object {
lateinit var instance: MyApplication
private set
}
// METHOD CALL
internal class ExampleNotificationHandler : NotificationHelperListener {
// FIRES WHEN A NOTIFICATION IS RECEIVED
override fun onNotificationReceived(payload: Payload) {
Log.i("iZootoExample", "payload = " + payload.title)
}
/*
1. If we clicked on notification then, it will show actionType 0.
2. If we clicked on button 1 then, it will show action type 1.
3. If we clicked on button 2 then, it will show action type 2.*/
// FIRES WHEN NOTIFICATION IS CLICKED
override fun onNotificationOpened(data: String) {
var actionType: String? = null
try {
val jsonObj = JSONObject(data)
actionType = jsonObj.getString("actionType")
if (actionType.equals("0")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
} else if (actionType.equals("1")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
} else if (actionType.equals("2")) {
val intent = Intent(instance, YOURACTIVITY::class.java)
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK)
instance.startActivity(intent)
}
} catch (e: JSONException) {
Log.e("TAG", "notificationOpened: " + e);
e.printStackTrace();
}
}
}
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate, iZootoNotificationActionDelegate {
func onNotificationReceived(payload: Aps) {
// Returns the content of Notification Payload.
print("Data",payload.alert?.title as Any)
}
}
FAQs
Why are the Call to Action Buttons Not Working?
When you get a notification with call to action buttons on mobile, you would need to pull down on it to see the buttons or swipe left and click View. On web, you will need to click the "Settings" button to see the drop down.
Make sure when you added the action button, you added all the required fields - ID, Title and Landing URL.
How can I Customise the Appearance of my App Push Call to Action Buttons?
This will require writing native Java code and manipulating the push notification layout using the Notification Service Extension.
To change the call to action button color, add something like the below with the Notification Service Extension:
// Example from Stackoverflow https://stackoverflow.com/questions/41073294/how-to-change-notification-action-text-color-in-android-n/56873390#56873390
Notification notification = new NotificationCompat.Builder(context, channelId)
...
.addAction(new NotificationCompat.Action.Builder(
R.drawable.ic_fire,
HtmlCompat.fromHtml("<font color=\"" + ContextCompat.getColor(context, R.color.custom_color) + "\">" + context.getString(R.string.fire) + "</font>", HtmlCompat.FROM_HTML_MODE_LEGACY),
actionPendingIntent))
.build())
.build();
If possible, you should modify the call to action button instead of using addAction
. Otherwise, you won't be able to define the button text from iZooto or track the click.
Why is there a close call to action button?
By default, web push notifications on Windows 10 include the Close button.
However, if you add your own call to action button, then this close button is removed. So, in either case the notification will remain on-screen till the user interacts with it.
This is designed by Google to give the users a chance to interact with the notification.
Updated almost 2 years ago