User Properties
You can use user properties to capture attributes such as demographic information or interests.
The demographic information may contain age, gender etc. Interests are usually derived attributes. If you already have this data against your users, you can onboard it on iZooto using user properties.
Capturing User Properties
User properties follow a key-value format, where key denotes the name of the user property and value refers to the value for an individual subscriber.
<script>
window._izq.push(["userProfile",{"add":{
"Gender":"Male" //Key - Value can be customized as per your requirements
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put("Gender","Male");
// Key: Value can be customized as per your requirement.
iZooto.addUserProperty(data);
let data = ["Gender": "Male"] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Gender": "Male"});
// For iOS
iZooto.addUserProperties("Gender","Male");
// For Android
iZooto.addUserProperty({"Gender": "Male"});
// For iOS
const obj = {"Gender": "Male"};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
It is important to note that, if a new value("FEMALE") is passed corresponding to the same key ("Gender", in this case), the value gets overwritten for the user from the previously categorized property("MALE") and would become part of the new value passed("FEMALE").
For app setup, make sure the token is set and SDK is properly initialized before passing user properties.
If you want to target users by the latest page that they visit, user properties would be the correct choice.
Example - You can add the following code on your website/app to capture the category of the last page visit for a user.
<script>
window._izq.push(["userProfile",{"add":{
"Category":"Sports"
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put("Category","Sports");
iZooto.addUserProperty(data);
let data = ["Category": "Sports"] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Category": "Sports"});
// For iOS
iZooto.addUserProperties("Category","Sports");
// For Android
iZooto.addUserProperty({"Category": "Sports"});
// For iOS
const obj = {"Category": "Sports"};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
Available Data Types
iZooto supports following data types for User Properties
1. String
This is the most commonly used Data type and comprises a set of characters that can also contain spaces and numbers.
<script>
window._izq.push(["userProfile",{"add":{
"Subscription Type":"Premium_User"
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put( "Subscription Type","Premium_User" );
iZooto.addUserProperty(data);
let data = [ "Subscription Type":"Premium_User" ] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Subscription Type": "Premium User"});
// For iOS
iZooto.addUserProperties("Subscription Type","Premium User");
// For Android
iZooto.addUserProperty({"Subscription Type": "Premium User"});
// For iOS
const obj = {"Subscription Type": "Premium User"};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
The filters available corresponding to String Data Type are:
- Equals
- Not Equals
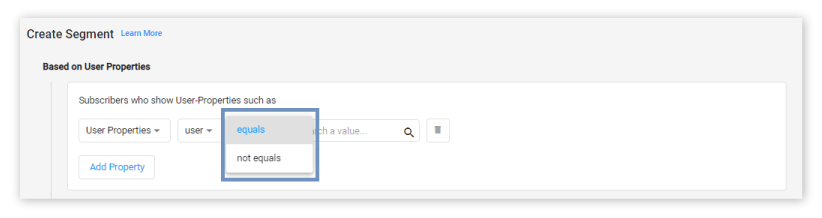
2. Number
This data type can be made use of if you wish to capture any numeric data against the user activity.
<script>
window._izq.push(["userProfile",{"add":{
"PinCode":10001
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put("Pincode",10001);
iZooto.addUserProperty(data);
let data = ["Pincode": 10001] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Pincode": 10001});
// For iOS
iZooto.addUserProperties("Pincode",10001);
// For Android
iZooto.addUserProperty({"Pincode": 10001});
// For iOS
const obj = {"Pincode": 10001};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
The filters available corresponding to Number Data Type are:
- Greater Than
- Less Than
- Equals
- Not Equals
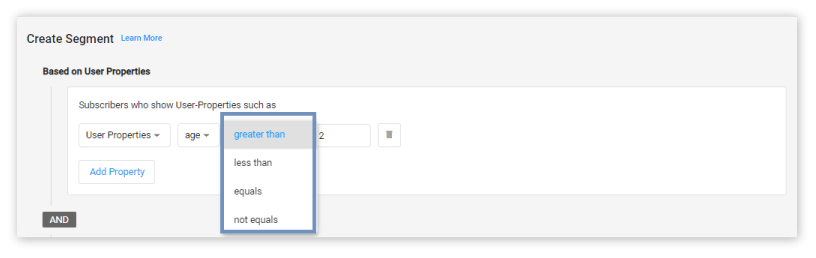
3. Boolean
Boolean accepts True or False as input and populates the relative filters based on that.
<script>
window._izq.push(["userProfile",{"add":{
"Sports_Visited": true
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put("Sports_Visited",true);
iZooto.addUserProperty(data);
let data = ["Sports_Visited": true] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Sports_Visited": true});
// For iOS
iZooto.addUserProperties("Sports_Visited",true);
// For Android
iZooto.addUserProperty({"Sports_Visited": true});
// For iOS
const obj = {"Sports_Visited": true};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
The filters available corresponding to Boolean Data Type are:
- Equals
- Not Equals
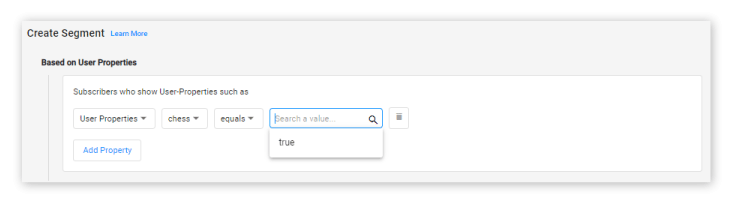
4. Date
The Date data type is useful if you want to capture and store certain date values against subscribers.
<script>
window._izq.push(["userProfile",{"add":{
"Date":"2018-08-23 03:04:05"
}
}]);
</script>
HashMap<String, Object> data = new HashMap<>();
data.put("Date","2018-08-23 3:04:05");
iZooto.addUserProperty(data);
let data = ["Date": "2018-08-23 3:04:05"] as [String : Any]
iZooto.addUserProperties( data: data)
// For Android
iZooto.addUserProperty({"Date": "2018-08-23 03:04:05"});
// For iOS
iZooto.addUserProperties("Date","2018-08-23 03:04:05");
// For Android
iZooto.addUserProperty({"Date": "2018-08-23 03:04:05"});
// For iOS
const obj = {"Date": "2018-08-23 03:04:05"};
const myJSON = JSON.stringify(obj);
iZooto.addUserProperty(myJSON);
The filters available corresponding to Date Data Type are:
Absolute:
- Before
- After
- On
- Between
Relative
- In The Past
- Was Exactly
- Today
- In The Future
- Will be exactly
- Within
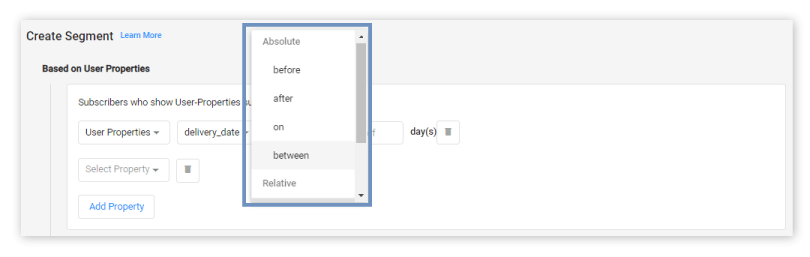
Predefined Properties
iZooto also captures a few additional properties by default that give us some basic information about the subscribers. Here are the properties:
Device:
The device they have subscribed from - Desktop, Mobile and Tablet
Device as a property is only available for Web Push Notifications.
Location:
The location of the device they have subscribed to. This can be used to send location-specific campaigns.
You will be able to drill down to the exact city of the subscriber.
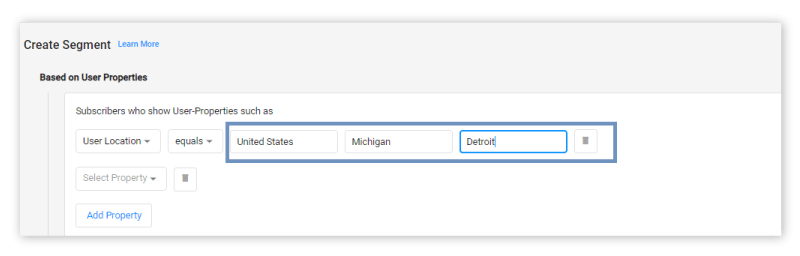
Subscription Date:
The date on which the subscription was captured.
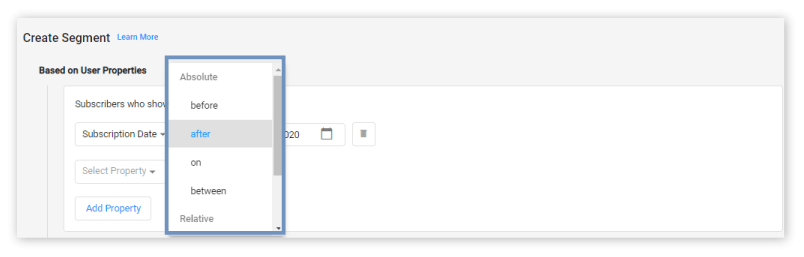
Capturing User Properties at the time of subscription
For Web Push Only
Often marketers want to capture specific attributes about the user such as language preference, country, website page from which the user subscribed and more.
While some of these attributes such as Subscription Date and Location are captured by default, other specific attributes can be collected by using the iZooto's callback function.
The properties can be captured at the time of subscription by using the register callback method
Here is how this works
- Language Preference
- Webpage from where the user subscribed
// Initializing iZooto
<script> window._izq = window._izq || []; window._izq.push(["init" ]); </script>
<script>
window._izq.push(["registerSubscriptionCallback",function(obj){
//These codes can be defined as per your needs. Refer to below table
if(obj.statuscode==1)
{
/*
Your code comes here
Extract language here, your javascript
var language =
*/
window._izq.push(["userProfile",{"add":{
"language":"en" /*whatever language you wish to pass*/
}
}]);
}
}]); </script>
//Domain specific JS.
<script src="https://cdn.izooto.com/scripts/83600dbdd34d91e3b153aaf01a445e32dc49e63b.js"></script>
After you successfully extract the language using iZooto’s Callback API as per the above example, segments basis different languages can be created.
Now when you are going to push a campaign, select the audience which you want the notification to be pushed to.
System Restrictions
-
The maximum no. of string properties that you can pass is 16 unique values.
-
Values for String type User-Properties support a maximum of 64 characters.
-
Data types supported are String, Numeric, Date, Boolean.
-
IMPORTANT The data type for existing User-Property will be modified as per the last entry. For instance, if you define a User-Property as 'Numeric', and pass a 'String' value to it, the data type will be changed to 'String', and operation filters in Audience Builder will be changed accordingly. You won't be able to see older data sent with the previous data type in 'Audience Builder' anymore.
-
Below mentioned are system properties reserved for internal use only. Please refrain from using these properties while defining user-properties at your end:
1.'_id'
2.'btype'
3.'pid'
4.'country'
5.'state'
6.'city'
7.'device’
8.'os'
9.'bver’
10.'ip'
11.'url’
12.'created_on'
13.'timezone'
14.'unique_id'
15.'auth_key'
16.'public_key’
17.'status'
18.'tags'
19.'auth_status'
20.'country_id'
21.'state_id'
22.'city_id'
23.'updated_on'
24.'bkey'
Updated 11 months ago